๐ ํด๋น ๊ฐ์ ๋ด์ฉ์ ์ฐธ๊ณ ํจ
C๋ก ๋ฐฐ์ฐ๋ ์๋ฃ๊ตฌ์กฐ ๋ฐ ์ฌ๋ฌ๊ฐ์ง ์์ ์ค์ต - ์ธํ๋ฐ | ํ์ต ํ์ด์ง
์ง์์ ๋๋๋ฉด ๋ฐ๋์ ๋์๊ฒ ๋์์ต๋๋ค. ์ธํ๋ฐ์ ํตํด ๋์ ์ง์์ ๊ฐ์น๋ฅผ ๋ถ์ฌํ์ธ์....
www.inflearn.com
์ฐ์ต1. ์ฌ์ฉ์ ์ ๋ ฅํ ๋ฌธ์์ด๊ณผ ๋ฌธ์์ด์ ๊ธธ์ด๋ฅผ ์ถ๋ ฅํ๊ธฐ
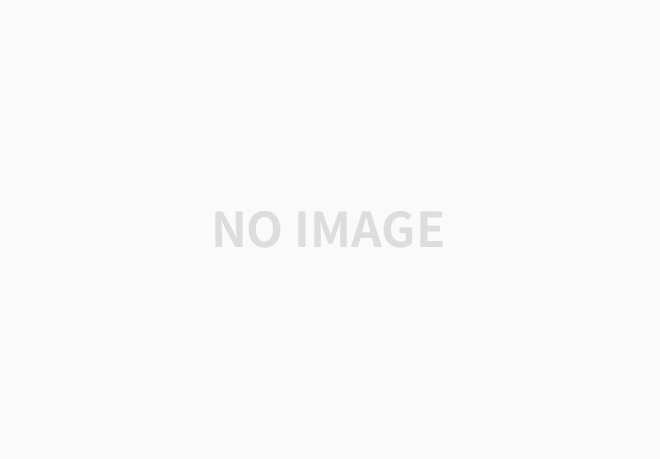
๋ฐฉ๋ฒ1) gets()
#include <stdio.h>
#include <string.h>
#define BUFFER_SIZE 20
int main(){
/*๋ฐฉ๋ฒ1 : gets์ฌ์ฉ - ์์ ํ์ง ์์*/
char input[BUFFER_SIZE];
while (1) {
printf("$ ");
gets_s(input, BUFFER_SIZE);
printf("%s:%d\n",input,strlen(input));
}
}
โพ BUFFER_SIZE๊น์ง๋ง ์ฝ์ด์ผ ํ๋๋ฐ ์ ํด์ง ๋ฒํผ์ ํฌ๊ธฐ๋ฅผ ์ด๊ณผํด์ ์ฝ์ -> ๋ฌธ์ ๊ฐ ๋จ
๋ฐฉ๋ฒ2) fgets()
/*๋ฐฉ๋ฒ2 : fgets์ฌ์ฉ - ๋ฌธ์ ์ ์๊ตฌ์ฌํญ์ ๋ถํฉํ์ง ์์*/
while (1) {
printf("$ ");
fgets(input, BUFFER_SIZE, stdin);
input[strlen(input) - 1] = '\0';
printf("%s:%d\n", input, strlen(input));
}
โพ ์ธ๋ฒ์งธ ๋งค๊ฐ๋ณ์๋ ํ์ผํฌ์ธํฐ, stdin๋ ํค๋ณด๋ ์
๋ ฅ
โพ ์ ํด์ง ๋ฒํผ์ ํฌ๊ธฐ๋ง ์ฝ์
โพ fgets๋ ์ํฐ๋ฅผ ์น๋ฉด \n๊น์ง ๋ฒํผ์ ์ ์ฅํ๊ธฐ ๋๋ฌธ์ ๋ฒํผ๋ฅผ ์ถ๋ ฅํ๋ฉด ์ค๋ฐ๊ฟ๊น์ง ์ถ๋ ฅ๋์ด๋ฒ๋ฆผ -> ๊ทธ๋์ ๋ง์ง๋ง ๋ฌธ์๋ฅผ \0๋ก ๋ณ๊ฒฝํ ๊ฒ
โพ ๋ฒํผ๋ฅผ ๋์ด์ ์ฝ์ด๋ฒ๋ฆผ -> ๋ฌธ์ ์ ์ทจ์ง์ ์ด๊ธ๋จ
๋ฐฉ๋ฒ3) getchar()
void main() {
/*๋ฐฉ๋ฒ3 : getchar๋ฅผ ์ฌ์ฉํ ์ฌ์ฉ์ ์ ์ ํจ์ ์ฌ์ฉ*/
while (1) {
printf("$ ");
read_line(input, BUFFER_SIZE);
printf("%s:%d\n", input, strlen(input));
}
}
int read_line(char str[], int limit) {
int ch, i = 0;
while ((ch = getchar()) != '\n') {
if (i < limit)
str[i++] = ch;
}
str[i] = '\0';
return i;
}
โพ getchar()์ ๋ฆฌํดํ์ ์ char๊ฐ ์๋๋ผ intํ์
โพ ๊ฐ์ฅ ์ ํํ ๋ฐฉ๋ฒ!
์ฐ์ต2. ์ฌ๋ฌ ์กฐ๊ฑด์ผ๋ก ๊ณต๋ฐฑ๋ฌธ์ ์ ๊ฑฐํ๊ธฐ
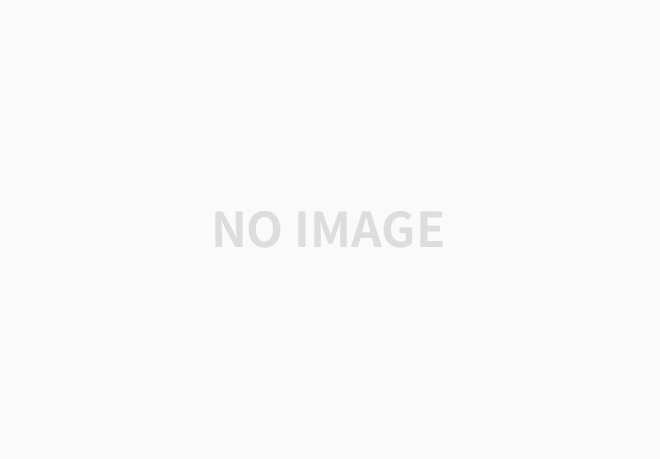
โพ ๋ฌธ์ ํด๊ฒฐ ์๋ฆฌ
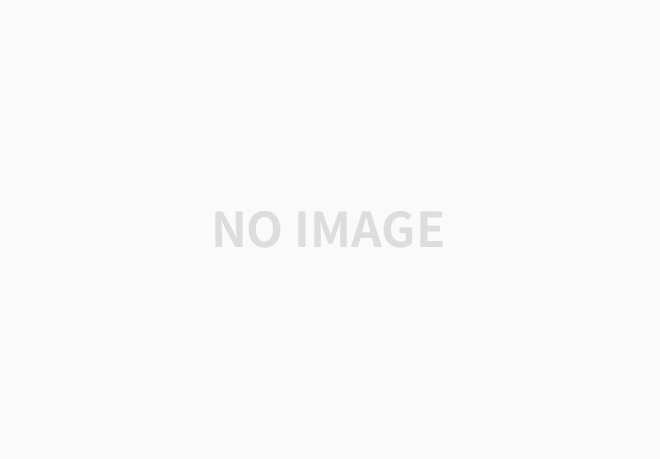
1) ๋ด๊ฐ ์์ฑํ ์ฝ๋
ํด์ค ๋ณด๊ธฐ ์ ์๋ ๋ชฐ๋๋๋ฐ ํด์ค๋ณด๊ณ ๋๋๊น compressed๋ฐฐ์ด์๋ ์ต๋ ํฌ๊ธฐ๋งํผ ์ ์ฅํ ์ ์๋๋ก ๋ง๋ค์์ด์ผ ํ๋๋ฐ ๊ทธ ๋ถ๋ถ์ด ๋น ์ ธ๋ฒ๋ ธ๋ค๋ ๊ฒ์ ์์๋ค... input์ ํฌ๊ธฐ ์ ์ฝ์ ๊ฑธ์ด๋จ๋ค...๐
#include <stdio.h>
#include <string.h>
#define BUFFER_SIZE 20
int main() {
char input[BUFFER_SIZE], compressed[BUFFER_SIZE];
while (1) {
printf("$ ");
int count = read_line(input, compressed, BUFFER_SIZE);
printf("%s:%d\n", compressed, count);
}
}
int read_line(char str[], char str2[], int limit) {
int ch, i = 0;
int count = 0;
while ((ch = getchar()) != '\n') {
if (i < limit)
str[i++] = ch;
}
str[i] = '\0';
for (int j = 0;j < strlen(str); j++) {
if (j != 0 && str[j] == ' ' && str[j - 1] != ' ') {
str2[count] = str[j];
count++;
}
else if (str[j] != ' ') {
str2[count] = str[j];
count++;
}
}
if (str2[count - 1] == ' ')
count--;
str2[count] = '\0';
return count;
}
2) ํด์ค
#include <stdio.h>
#include <string.h>
#include <ctype.h>
#define BUFFER_SIZE 20
int main() {
char line[BUFFER_SIZE];
while (1) {
printf("$ ");
int count = read_line_with_compression(line, BUFFER_SIZE);
printf("%s:%d\n", line, count);
}
return 0;
}
int read_line_with_compression(char compressed[], int limit) {
int ch, i = 0;
while ((ch = getchar()) != '\n') {
if (i < limit - 1 && (!isspace(ch) || i > 0 && !isspace(compressed[i - 1])))
// !isspace(ch) : ๊ณต๋ฐฑ์ด ์๋๊ฑฐ๋
// i > 0 && !isspace(compressed[i - 1]) : ๊ณต๋ฐฑ์ด์ง๋ง ์ง์ ์ ๋ฌธ์๊ฐ ๊ณต๋ฐฑ์ด ์๋ ๊ฒฝ์ฐ
compressed[i++] = ch;
}
if (i > 0 && isspace(compressed[i - 1]))
i--;
compressed[i] = '\0';
return i;
}
โพ <ctype.h>์ isspace() ํจ์๋ฅผ ์ฌ์ฉ
โพ isspace(ch)์ ch!=' '์ ๊ฐ์
'Language > C++' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
[C์๋ฃ๊ตฌ์กฐ] ์ ํ๋ฒํธ๋ถ1 - ์์์ ๊ด๊ณ์์ด ๋ฐฐ์ด๋ก CRUD๊ตฌํ (0) | 2021.08.01 |
---|---|
_CRT_SECURE_NO_WARNINGS ์ค๋ฅ (0) | 2021.03.06 |
[C] ๊ตฌ์กฐ์ฒด(struct)์ ๊ณต์ฉ์ฒด(union) (0) | 2021.02.25 |
๋๊ธ